Top 5 JavaScript Questions on Stack Overflow
Stack Overflow is the world's largest community of developers. On a typical day, developers ask over 8,000 questions on the platform about programming problems they run into. In this article, we're taking a look at the top 5 most popular JavaScript questions and their solutions with the help of ChatGPT.
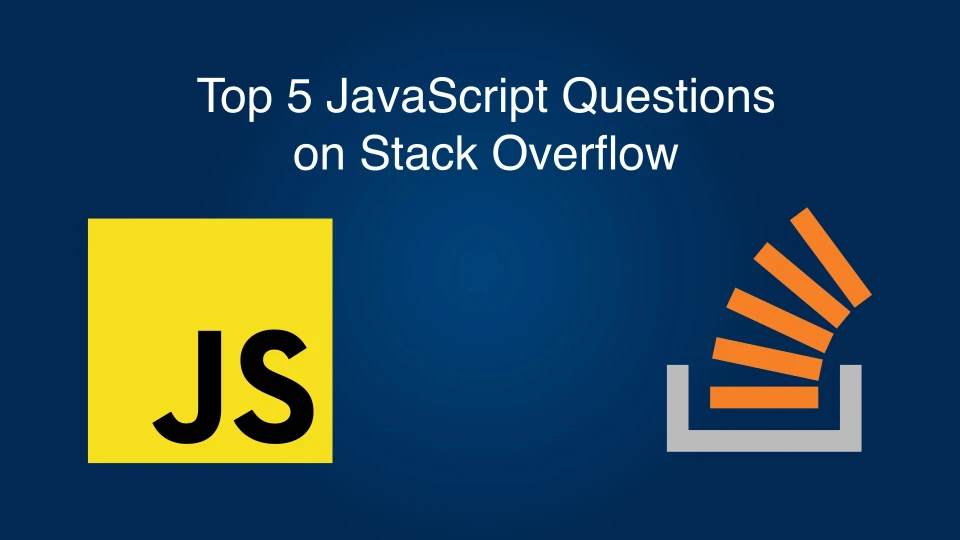
5. How to check if one text string includes text string?
Using
String.prototype.includes
method.
This method returns true
if the original string contains the specified string and false
otherwise.
const mainString = "The quick brown fox jumps over the lazy dog.";const searchString = "fox"; if (mainString.includes(searchString)) { console.log("The main string includes the searched string.");} else { console.log("The main string does NOT include the searched string.");}
4. How to redirect to another page?
Using
window.location
object.
// Redirect to another pagewindow.location.href = "https://www.khalidcodes.com"; // Orwindow.location.assign("https://www.khalidcodes.com"); // Redirect and replace current page in history.// In other words, user CANNOT use the back button to go to the previous url.window.location.replace("https://www.khalidcodes.com");
3. What does 'use strict' do?
The statement
"use strict";
is a directive that enables a stricter set of parsing and error handling rules in the JavaScript code.
When you use strict mode, the JavaScript interpreter enforces a more rigorous set of rules, which helps to catch common coding mistakes and prevent the use of certain error-prone features.
Some key characteristics of strict mode include:
- Error reporting: In strict mode, certain common coding errors that could otherwise go unnoticed or fail silently will throw errors. This helps developers identify and fix issues early in the development process.
- Variables: Variables must be declared with
var
,let
, orconst
before being used. In non-strict mode, undeclared variables are automatically global, which can lead to unintended consequences. - No implied global variables: Assigning a value to an undeclared variable, as well as assigning a value to
this
outside of a function or a constructor, will result in an error.
To use strict mode, you only need to include the following line at the beginning of a script or a function:
"use strict";
2. How to know if an element is visible?
It is challenging to create a one-size-fits-all solution that works in all cases. But generally, we can check the element's CSS properties to cover the most common situations.
// Check the element's propertiesconst isVisible = ( el.style.display !== "none" && el.visibility !== "hidden"); // Check the element's positionconst position = el.getBoundingClientRect();const isInView = ( position.top >= 0 && position.bottom <= window.innerHeight);
We can also determine if an element is visible by checking its offsetWidth
and offsetHeight
properties, along with the getComputedStyle
function.
function isElementVisible(element) { // Get the computed style of the element let style = window.getComputedStyle(element); // Check if the element is both displayed and has non-zero dimensions return style.display !== 'none' && style.height !== '0' && style.width !== '0';} // Example usage:let myElement = document.getElementById("exampleElement");if (isElementVisible(myElement)) { console.log("The element is visible.");} else { console.log("The element is not visible.");}
The function isElementVisible()
checks if the element is not set to display: none
and has non-zero height and width. Keep in mind that this method doesn't account for elements hidden behind other elements or those with visibility set to hidden. Depending on your specific use case, you might need a more sophisticated approach.
1. How to remove a specific element from an array?
There are multiple methods you can use to remove an element from an array in JavaScript. Here are a couple of common approaches:
- The
splice()
method changes the contents of an array by removing or replacing existing elements and/or adding new ones.
let array = [1, 2, 3, 4, 5];let elementToRemove = 3; // Find the index of the elementlet index = array.indexOf(elementToRemove); // Check if the element is present in the arrayif (index !== -1) { // Remove the element using splice array.splice(index, 1);} console.log(array); // Output: [1, 2, 4, 5]
- The
filter()
is another method that creates a new array with all the elements that pass the test provided by a function.
let array = [1, 2, 3, 4, 5];let elementToRemove = 3; // Use filter to create a new array without the element to removearray = array.filter(item => item !== elementToRemove); console.log(array); // Output: [1, 2, 4, 5]
Both methods achieve the goal of removing a specific element from an array. Choose the one that fits your use case and coding style preference.
Until next time. ✌️