How to convert a callback function to an async/await function
In JavaScript, async/await is a feature that provides a more concise and readable way to work with asynchronous code. It's built on top of promises and provides a way to write asynchronous code that looks and behaves more like synchronous code.
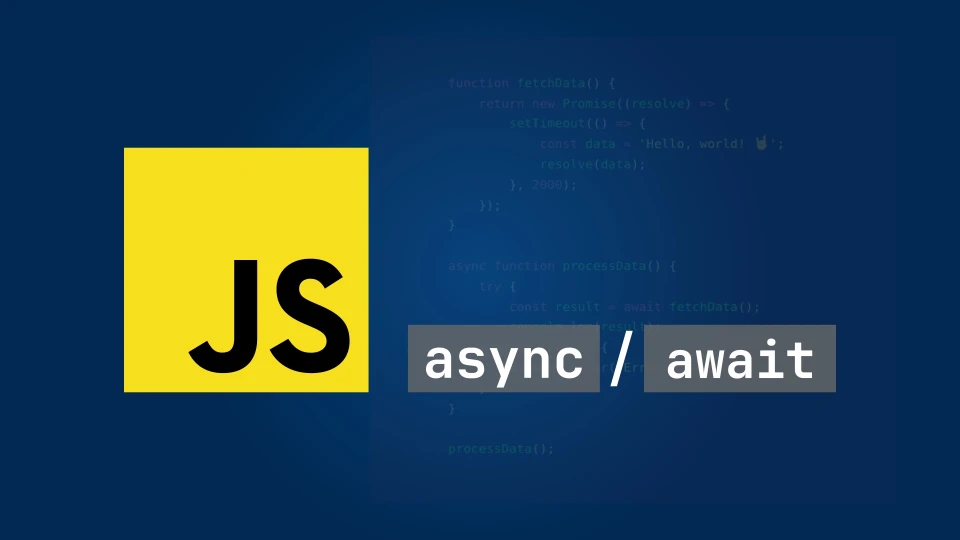
Synchronous Code
In synchronous code, each operation is executed one after the other in a sequential manner. Each operation must complete before the next one starts. If there is a task that takes time to complete, the entire program will be blocked, and no other code will execute until that task is finished.
Here is an example:
console.log('Start'); function synchronousOperation() { for (let i = 0; i < 3; i++) { console.log('Sync operation', i); }} synchronousOperation(); console.log('End');
In this example, the synchronousOperation function runs in a blocking manner, and the "End" message is only logged after it completes.
Asynchronous Code
In asynchronous code, tasks can be initiated, and the program can continue to execute other operations without waiting for those tasks to finish. Callbacks, Promises, and the async/await syntax are common ways to work with asynchronous code in JavaScript.
Here's an asynchronous example using callbacks:
console.log('Start'); function asynchronousOperation(callback) { setTimeout(() => { console.log('Async operation'); callback(); }, 2000);} asynchronousOperation(() => { console.log('Callback executed');}); console.log('End');
In this example, the asynchronousOperation function initiates an asynchronous task (a setTimeout in this case) and accepts a callback function. While the asynchronous task is running, the program continues to execute, and the "End" message is logged before the asynchronous task completes.
Asynchronous code is crucial for handling tasks that might take time to complete, such as network requests, file I/O, or other operations that don't happen instantly. It helps prevent blocking and keeps the program responsive.
async/await
In JavaScript, async/await
is a feature that provides a more concise and readable way to work with asynchronous code. It's built on top of promises and provides a way to write asynchronous code that looks and behaves more like synchronous code.
If you're not familiar with this feature, here's a basic breakdown:
Async Function
- An
async
is a function that always returns a promise. - The
async
keyword is placed before the function declaration.
async function myAsyncFunction() { // Async code goes here}
Await Operator
- The
await
keyword can only be used inside an async function. - It pauses the execution of the function until the promise is resolved and returns the resolved value.
async function myAsyncFunction() { const result = await someAsyncOperation(); // Code here executes after someAsyncOperation is complete console.log(result);}
An example of how async/await
can be used to handle asynchronous operations would be something like:
function fetchData() { return new Promise((resolve) => { setTimeout(() => { const data = 'Hello, world! 🤘'; resolve(data); }, 2000); });} async function processData() { try { const result = await fetchData(); console.log(result); } catch (error) { console.error('Error:', error); }} processData();
In this example, fetchData()
returns a promise, and processData()
is marked as an async
function. The await
keyword is used to wait for the promise to resolve before moving on to the next line of code.
This makes asynchronous code look more like synchronous code, improving readability and maintainability.
Hope this was helpful, until next time. ✌️